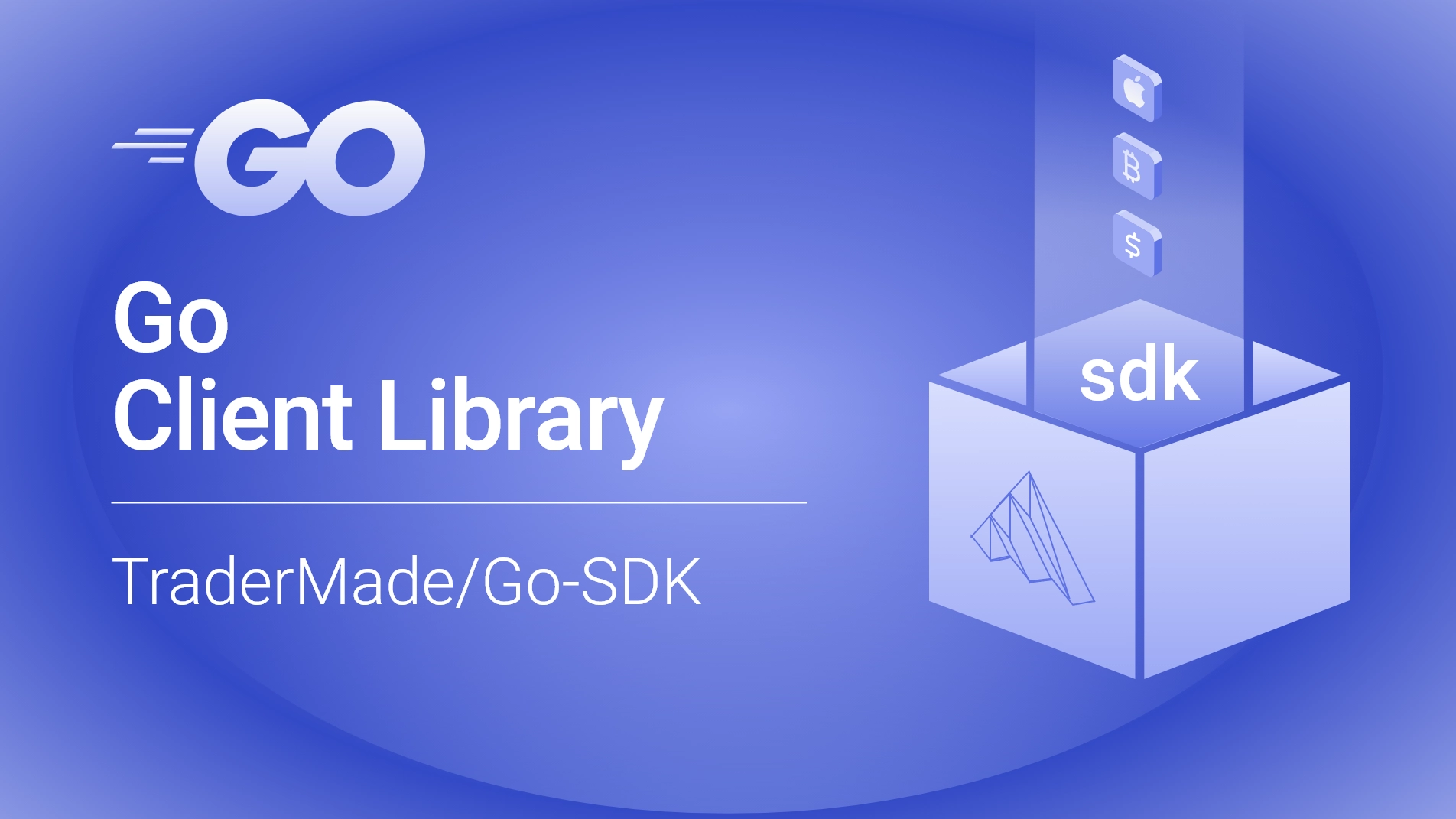
Accessing Market Data with TraderMade’s Go Library
16 October 2024
This tutorial demonstrates how to access currency exchange rates, precious metals, and crypto prices using TraderMade's Go Library. TraderMade is a financial data platform. It offers real-time and historical market data. You can find Forex, Crypto, Stocks, Precious Metals, and Energy CFDs data. With Our Client Go library, developers, traders, and product managers can quickly integrate API into their projects.
Getting Started
To access real-time and historical Forex market data with TraderMade, initially create an account and get an API key for authentication. Explore more regarding various types of market data offered, reference data, and available real-time streaming data by going through the TraderMade API documentation thoroughly.
To get started, please make sure you're running at least Go 1.21+ for module support. You'll need to get the tradermade/Go-SDK client library:
go get github.com/tradermade/Go-SDK
From here, interacting with the TraderMade API is easy. Let's look at some example code snippets to help you get started.
Initializing the REST Client
import ( tradermade "github.com/tradermade/Go-SDK/rest" ) client := tradermade.NewRESTClient("YOUR_API_KEY")
Check out stocks, indices, energy, & precious metals we cover: Supported CFDs
Also, learn more about our:
1) Forex API
2) CFD API
3) Crypto API
Example: Fetching Live Forex Rates
Here's how to fetch live Forex rates for Gold and EURUSD:
currencyPairs := []string{"EURUSD", "XAUUSD"} liveRates, err := client.GetLiveRates(currencyPairs) if err != nil { log.Fatalf("Error fetching live rates: %v", err) } for _, quote := range liveRates.Quotes { fmt.Printf("Base: %s, Quote: %s, Bid: %f, Ask: %f, Mid: %f ", quote.BaseCurrency, quote.QuoteCurrency, quote.Bid, quote.Ask, quote.Mid) }
This code snippet demonstrates how to:
1) Initialize the TraderMade REST client with your API key.
2) Define a list of currency pairs you want to fetch data for.
3) Use the GetLiveRates method to fetch live Forex rates.
4) Handle potential errors.
5) Print the results, including the base currency, quote currency, bid price, ask price, and mid-price.
Example: Currency Conversion
TraderMade also provides live currency conversion. Here's how to fetch currency conversion in real-time:
convertResult, err := client.ConvertCurrency("EUR", "GBP", 1000.0) if err != nil { log.Fatalf("Error fetching conversion data: %v", err) } fmt.Printf("Converted %s to %s: ", convertResult.BaseCurrency, convertResult.QuoteCurrency) fmt.Printf("Quote: %f ", convertResult.Quote) fmt.Printf("Total: %f ", convertResult.Total) fmt.Printf("Requested Time: %s ", convertResult.RequestedTime) fmt.Printf("Timestamp: %d ", convertResult.Timestamp)
This example shows how to:
1) Use the same REST client to fetch currency conversion.
2) Specify a currency pair, date, and interval (in this case, daily data).
3) Use the ConvertCurrency method to fetch the data.
4) Handle and print the results, including open, high, low, and close prices.
Example: Hourly Timeseries Data
TraderMade also provides historical data useful to visualize data. For example, you can draw an intraday chart or calculate pivots. Here's how to fetch timeseries data:
timeSeriesDataHourly, err := client.GetTimeSeriesData("EURUSD", "2024-10-01 10:00", "2024-10-02-11:00" , "hourly", 4) if err != nil { log.Fatalf("Error fetching hourly time series data: %v", err) } fmt.Printf("Time Series Data (Hourly) from %s to %s: ", timeSeriesDataHourly.StartDate, timeSeriesDataHourly.EndDate) for _, quote := range timeSeriesDataHourly.Quotes { fmt.Printf("Date: %s, Open: %f, High: %f, Low: %f, Close: %f ", quote.Date, quote.Open, quote.High, quote.Low, quote.Close) }
Example: Real-time Forex Data with WebSocket
For continuous data streaming for algo trading or to build a quotes board, it's great to use WebSockets.
You would need a WebSocket Key (not the same as API key) - You can start a free trial for up to 14 days.
Import the WebSocket client package to get started.
import ( tradermadews "github.com/tradermade/Go-SDK/websocket" )
Next, create a new client with your WebSocket API key and currency pairs.
// Initialize the WebSocket client with your API key client := tradermadews.NewWebSocketClient("YOUR_WS_KEY", "EURUSD,GBPUSD,XAUUSD,SPX500USD")
The Library allows you to reconnect if the connection is closed. You can customize the number of tries and retry intervals according to your needs.
// Set custom retry settings client.MaxRetries = 10 // Set maximum number of retries client.RetryInterval = 5 * time.Second // Set retry interval // Enable automatic reconnection client.EnableAutoReconnect(true) // Connect to the TraderMade WebSocket err := client.Connect() if err != nil { log.Fatal(err) } defer client.Disconnect() // Ensure to disconn
The client automatically reconnects to the server when the connection is dropped. When the client successfully reconnects, it resubscribes to the symbols set during initialization.
Using the client
After creating a client, set up handlers for different events and start receiving data.
// Set a handler for the "Connected" message client.SetConnectedHandler(func(connectedMsg tradermadews.ConnectedMessage) { fmt.Printf("WebSocket connected: %s ", connectedMsg.Message) }) // Set a message handler to process received quotes client.SetMessageHandler(func(quote tradermadews.QuoteMessage, humanTimestamp string) { fmt.Printf("Received quote: Symbol=%s Bid=%.5f Ask=%.5f Timestamp=%s (Human-readable: %s) ", quote.Symbol, quote.Bid, quote.Ask, quote.Ts, humanTimestamp) }) // Set a handler to notify reconnection attempts client.SetReconnectionHandler(func(attempt int) { fmt.Printf("Reconnecting... (Attempt %d) ", attempt) }) // Handle graceful shutdown (Ctrl+C) c := make(chan os.Signal, 1) signal.Notify(c, syscall.SIGINT, syscall.SIGTERM) <-c fmt.Println("Shutting down WebSocket client...")
This example demonstrates how to:
1) Initialize the WebSocket client with your API key and the currency pairs you want to subscribe to.
2) Set custom retry settings and enable automatic reconnection.
3) Set up handlers for different events (connection, message reception, reconnection attempts).
4) Connect to the WebSocket and start receiving real-time currency, stock indices and precious metals data.
5) Handle graceful shutdown when the program is interrupted.
The WebSocket client offers a significant advantage by delivering instantaneous updates, making it an excellent choice for applications that demand up-to-the-minute Forex information.
Conclusion
The TraderMade Go SDK simplifies the process of accessing Forex market data by providing a user-friendly interface that supports both REST API and WebSocket connections. With minimal coding effort, you can retrieve current rates and historical data, perform currency conversions, and even receive real-time Forex data streams.
By utilizing TraderMade's comprehensive Forex Data API and the Go SDK, developers can create robust financial applications, trading systems, and analytical tools. Whether you need historical data for backtesting or real-time data for live trading, TraderMade's Go SDK is a versatile solution.
For in-depth information and practical examples, please refer to the TraderMade Go SDK documentation and the GitHub repository.