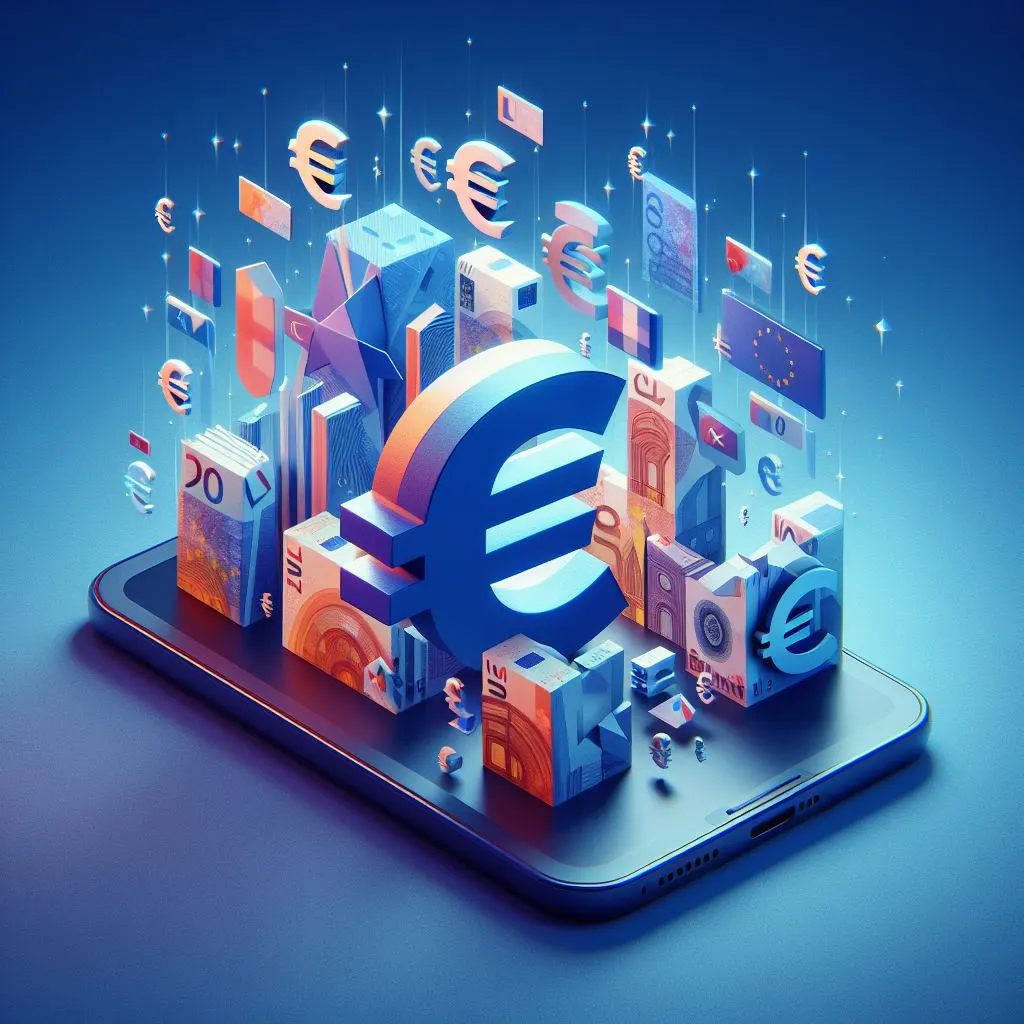
Build Your First Currency Converter in Python
16 May 2024
Dealing with foreign exchange and currency conversion has become part of the routine for many developers working in the fintech space, and analyzers help others make informed decisions. However, getting the currency conversion right is crucial, as currency exchange rates fluctuate. How about building your currency converter app that can be shared with colleagues and teams using real-time currency exchange rates? Sounds interesting?
You may wonder what it is about and how to develop one. The following tutorial provides easy yet crucial steps that can simplify the process.
What is a currency converter app?
The currency converter application is a tool that helps users precisely convert one currency's price to another's. The best part is that conversions happen real quick, within milliseconds. Users can keep track of continuously fluctuating rates with little effort.
How can you share it with others?
Building a currency converter app is just one part of the puzzle other is to share it with others, though this is easy for experienced programmers if you need to do it even as a beginner then Streamlit library is a quick and easy place to start.
What is Streamlit?
Streamlit is an open-source Python library. It enables developers to build attractive user interfaces. It is one of the easiest ways to put code in a web application, especially when people do not know the front end. The best part of Streamlit is that you do not need to learn the basics of web development to create an app. Hence our choice to build this currency conversion app. Now that we have a basic understanding of our application let's look at building one.
What is needed to build a Currency Converter App?
Currency Converter App requires largely three things: API to get Currency Rates, basic programming knowledge, and a Streamlit account to deploy your app.
How to make a Currency Converter in Python?
To build the app, we need to sign up to an API and install Python. Once these two things are ready we will open a terminal and install the Streamlit Library.
pip install streamlit
Once installed, we are ready to build a Python Currency Converter. We will start our Forex Python program by importing the libraries needed to get rates and display them on the web without writing the front-end code.
import streamlit as st import requests
To acquire the required foreign exchange rates, we will define a base URL and the API key. The URL calls the convert endpoint and makes it fairly easy to convert from one currency to another.
base_url = " https://marketdata.tradermade.com/api/v1/convert" api_key = "API_KEY" # Replace 'api_key' with your actual API key
To display a logo or an image, save it in the same directory as the code file and set the image using the following function. Feel free to edit it accordingly.
st.image("tradermade_logo-01.png", width=200)
To make it manageable we will define a convert currency function that will take in three parameters; namely amount, from_currency, and to_currency. The Python function will return the currency conversion rate and the total converted amount.
Here, the url, f"{base_url}?api_key={api_key}&from={from_currency}&to={to_currency}&amount={amount}", is formed by combining base URL and API key and the three params we mentioned above .
# Define function to convert currency def convert_currency(amount, from_currency, to_currency): url = f"{base_url}?api_key={api_key}&from={from_currency}&to={to_currency}&amount={amount}" response = requests.get(url) if response.status_code == 200: rate = response.json()["quote"] print(rate) converted_amount = response.json()["total"] return rate, converted_amount else: return None, None
The API provides tens of currencies which amount to over 4000+ pairs hence we will define a function that requests the currencies provided and use that to form a dropdown list for easy selection later.
def fetch_supported_currencies(): url = "https://marketdata.tradermade.com/api/v1/live_currencies_list?api_key=API_KEY" response = requests.get(url) print(response) # Check if the request was successful if response.status_code == 200: currencies_data = response.json() if "available_currencies" in currencies_data: currencies = currencies_data["available_currencies"] return list(currencies.keys()) else: st.write("Error: 'available_currencies' key not found in response.") return None else: st.write(f"Error {response.status_code}: {response.text}") return None
The final function we define will be a Currency Converter. The function takes in a user input as an integer amount. The function also asks the user to select a “from” and “to” currency from a list of supported currencies fetched from the above function. The second select statement is a multi-select that lets the user select more than one currency at a time. Finally, the ‘convert’ button is rendered, which fetches the rates to show the currency conversion.
# Define the Streamlit app for currency conversion def currency_converter(): # Input amount amount = st.number_input("Enter an integer amount to convert:", value=100, step=1) # Fetch all supported currencies from the API supported_currencies = fetch_supported_currencies() if supported_currencies is not None: # Input 'from' currency from_currency = st.selectbox("From currency:", supported_currencies,index=19) # Input 'to' currencies to_currencies = st.multiselect("To currencies:", supported_currencies, default=["USD"]) # Convert currency and display result if st.button("Convert"): st.write("Conversion results:") for to_currency in to_currencies: print(to_currency, from_currency) try: rate, converted_amount = convert_currency(amount, from_currency, to_currency) if rate: st.write(f"{amount} {from_currency} = {converted_amount} {to_currency} - 1{from_currency} = {rate} {to_currency}") else: st.write(f"Something Went Wrong Please Check with your API Provider") except: st.write(f"Error: Exchange rates not available for {from_currency} to {to_currency}.")
The whole file runs as a server which, can be deployed on your local computer or the Streamlit Website.
# Run the Streamlit app if __name__ == "__main__": currency_converter()
To start the server save the above file as currency_converter.py The following code helps to execute the code.
streamlit run currency_converter.py
Output
Hurray! We have our output. We have displayed currency exchange rates both the per unit rates and the total amount, you can do as you need, including creating an inverse.
Conclusion
We have built one of the simplest apps to convert currency from one to multiple with the help of live FX rates. Feel free to customize it accordingly. Our Currency Converter app is free to use.
If you need assistance, please don't hesitate to contact us via live chat or email support@tradermade.com.