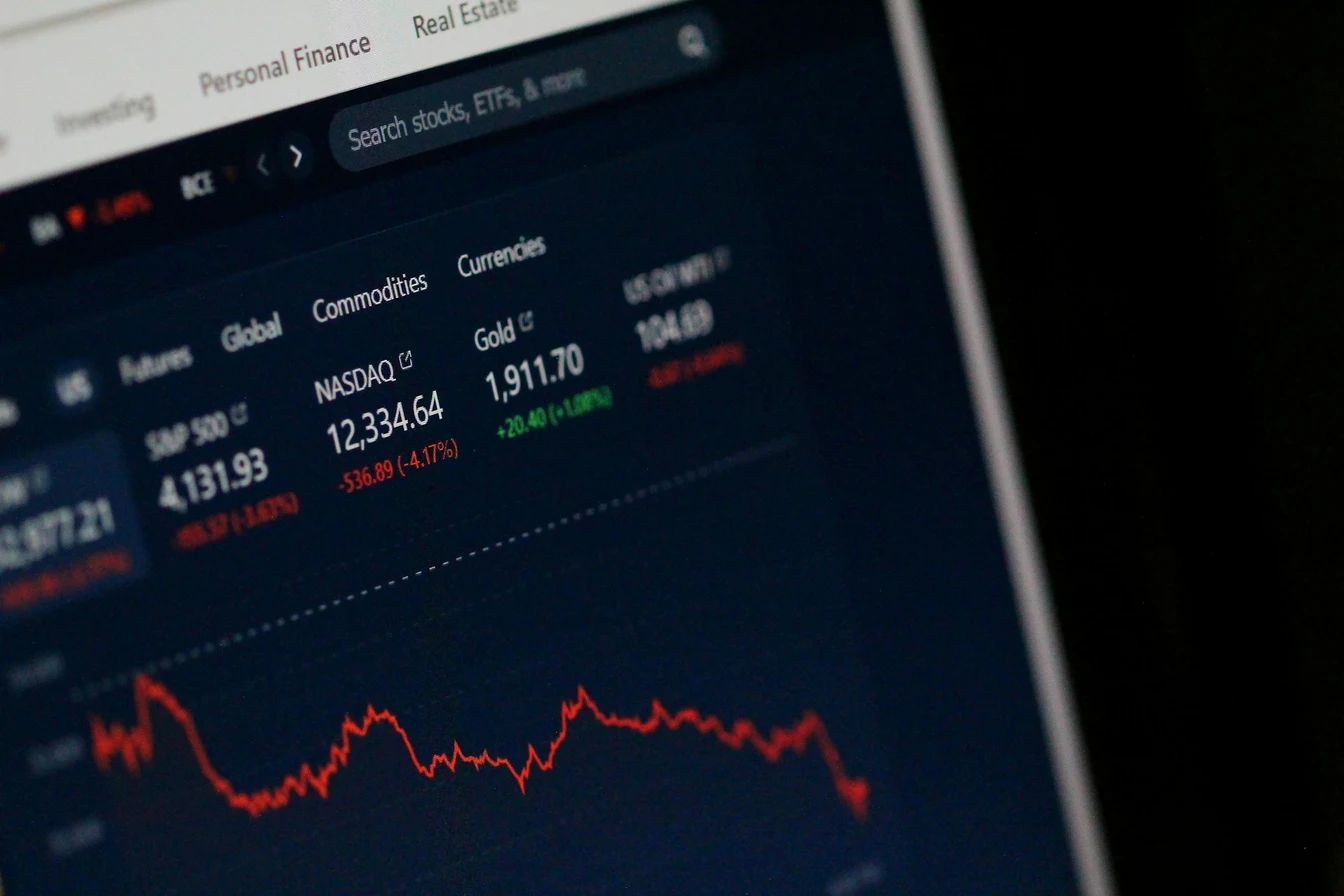
Get Live Gold And Silver Prices Instantly with Precious Metals API
13 June 2024
In today's dynamic financial landscape, tracking real-time precious metal prices is crucial for investors, developers, and enthusiasts alike. In this tutorial, you'll learn how to get gold and silver prices via the precious metals API.
Whether you're an expert or just starting, this guide provides the tools to access accurate and timely data. It offers a detailed overview of accessing spot pricing for precious metals data using TraderMade’s REST API with various programming languages.
Why Precious Metals Data Matter
For centuries, precious metals have been valued for their rarity and intrinsic worth. Accurate precious metals pricing data is crucial for investment, manufacturing, jewelry, and financial analysis. Understanding how to receive and interpret data from the Precious Metals Prices API is key to making informed decisions across these industries.
Methods to Receive Real-Time Financial Data
APIs
What is an API? An Application Programming Interface (API) allows you to access real-time financial data from different platforms programmatically.
1) Sign Up: Register for an API key on the provider's website.
2) Choose a Plan: Select a free or paid plan based on your data needs.
3) Access Data: Use the API key to request data through your preferred programming language (e.g., Python, JavaScript).
Python Programming
This Python script utilizes the requests library to fetch live Gold price via API. It also fetches silver and platinum prices in the same request as shown below.
import requests from pprint import PrettyPrinter url = "https://marketdata.tradermade.com/api/v1/live" currency = "XAUUSD,XAGUSD,XPTUSD,XPDUSD" api_key = "API_KEY" # Replace with your actual API key querystring = {"currency": currency, "api_key": api_key} response = requests.get(url, params=querystring) # Check if the request was successful if response.status_code == 200: pp = PrettyPrinter() pp.pprint(response.json()) else: print(f"Error {response.status_code}: {response.text}")
Java Programming
This Java program requests data from the API for Gold prices using an HTTP GET request. It can also be extended to obtain silver price data and other live precious metal prices. The "FetchMarketData" class defines the API URL and parameters, including a list of currency codes and an API key.
import org.apache.http.HttpEntity; import org.apache.http.HttpHeaders; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import org.apache.http.util.EntityUtils; import java.io.IOException; import org.json.JSONArray; import org.json.JSONObject; public class RESTClient { public static void main(String[] args) throws IOException { CloseableHttpClient httpClient = HttpClients.createDefault(); try { HttpGet request = new HttpGet("https://marketdata.tradermade.com/api/v1/live currency=XAUUSD&api_key=YOUR_API_KEY"); CloseableHttpResponse response = httpClient.execute(request); try { HttpEntity entity = response.getEntity(); if (entity != null) { // return it as a String String result = EntityUtils.toString(entity); System.out.println(result); JSONObject obj = new JSONObject(result); JSONArray quotes = obj.getJSONArray("quotes"); System.out.println(quotes.toString()); for (int i = 0; i < quotes.length(); i++) { JSONObject quote = quotes.getJSONObject(i); System.out.println(" Quote " + quote.getString("base_currency") + quote.getString("quote_currency") + " " + quote.getFloat("bid") + " " + quote.getFloat("ask")); } } } finally { response.close(); } } finally { httpClient.close(); } } }
Node.js Programming
This Node.js script utilizes the Axios library to fetch real-time Gold Price data via the live API endpoint.
const axios = require('axios'); const util = require('util'); const url = "https://marketdata.tradermade.com/api/v1/live"; const currency = "XAUUSD"; const apiKey = "API_KEY"; // Replace with your actual API key async function fetchMarketData() { try { const response = await axios.get(url, { params: { currency: currency, api_key: apiKey } }); if (response.status === 200) { console.log(util.inspect(response.data, { depth: null, colors: true })); } else { console.log("Error"); } } catch (error) { console.error("Error"); } } fetchMarketData();
C++ Programming
This C++ code snippet demonstrates how to fetch data from the live API endpoint to get Gold price using the libcurl library. The code initiates by including the necessary headers for libcurl functions and standard input/output operations.
#include <curl/curl.h> #include <stdio.h> int main(void) { CURL *curl; CURLcode res; // URL to be requested const char *url = "https://marketdata.tradermade.com/api/v1/live?currency=XAUUSD&api_key=API_KEY"; // Initialize global curl environment curl_global_init(CURL_GLOBAL_DEFAULT); // Initialize curl session curl = curl_easy_init(); if(curl) { // Set URL curl_easy_setopt(curl, CURLOPT_URL, url); // Perform the request res = curl_easy_perform(curl); // Check for errors if(res != CURLE_OK) { fprintf(stderr, "Request failed: %s ", curl_easy_strerror(res)); } else { printf("Request was successful! "); } // Cleanup curl session curl_easy_cleanup(curl); } // Cleanup curl global environment curl_global_cleanup(); return 0; }
Conclusion
This tutorial provides an overview of accessing and utilizing precious metals data through JSON API using various programming languages. By leveraging these methods, you can effectively integrate live market data using our live API endpoint for Gold, Silver, Platinum, and Palladium prices, into your applications, enhancing your ability to incorporate them into your apps and workflow. Refer to our documentation page for examples in other programming languages and information on live and historical rates for world currencies.