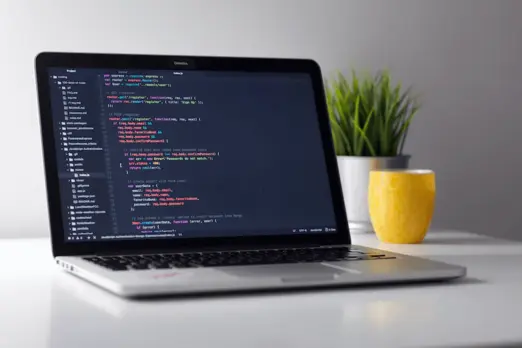
Real-Time Forex and CFD Data with C# and FIX
09 January 2023
This tutorial is a guide to setup your Visual Studio Code environment and developing a program to request Live Forex Data from Trademades FIX service.
I will assume you have Visual Studio Code and .Net 7.0 installed on your computer.
First, let's set up the environment
Open Visual Studio Code.
Select File > Open Folder (File > Open... on macOS) from the main menu. In the Open Folder dialog, create a CSHARPFIX folder and select it. Then click Select Folder (Open on macOS).
The project name and the namespace will be called CSHARPFIX by default. You'll add code later in the tutorial that assumes the project namespace is CSHARPFIX
Next, you will see a dialog saying “Do you trust the authors of the files in this folder?”, select “Yes, I trust the authors.”
Open the Terminal in Visual Studio Code by selecting View > Terminal from the main menu. The Terminal opens with the command prompt in the CSHARPFIX folder.
Setup the .NET framework for your project
In the Terminal, enter the following command:
dotnet new console --framework net7.0
Now we can import the QuickFix libs:
dotnet add package QuickFIXn.FIX4.4 --version 1.10.0
By default the projected template will be created with a simple hello world program. Remove this and replace it with the example code below:
using QuickFix; using QuickFix.Fields; public class MyQuickFixApp : IApplication { public void FromApp(Message msg, SessionID sessionID) { System.Console.WriteLine(" From App " + msg); } public void OnCreate(SessionID sessionID) { } public void OnLogout(SessionID sessionID) { } public void OnLogon(SessionID sessionID) { System.Console.WriteLine(" OnLogon "); QuickFix.FIX44.MarketDataRequest msg = new QuickFix.FIX44.MarketDataRequest(); // Fill message fields msg.SetField(new MDReqID("123")); msg.SetField(new SubscriptionRequestType('1')); msg.SetField(new MarketDepth(0)); msg.SetField(new MDUpdateType(0)); // Add the MDEntryTypes group QuickFix.FIX44.MarketDataRequest.NoMDEntryTypesGroup noMDEntryTypes = new QuickFix.FIX44.MarketDataRequest.NoMDEntryTypesGroup(); noMDEntryTypes.SetField(new MDEntryType('0')); msg.AddGroup(noMDEntryTypes); // Add the NoRelatedSym group QuickFix.FIX44.MarketDataRequest.NoRelatedSymGroup noRelatedSym = new QuickFix.FIX44.MarketDataRequest.NoRelatedSymGroup(); noRelatedSym.SetField(new Symbol("GBPUSD")); msg.AddGroup(noRelatedSym); // Send message Session.SendToTarget( msg, sessionID); } public void FromAdmin(Message msg, SessionID sessionID) { System.Console.WriteLine(" From Admin " + msg); } public void ToAdmin(Message msg, SessionID sessionID) { System.Console.WriteLine(" To Admin " + msg); } public void ToApp(Message msg, SessionID sessionID) { System.Console.WriteLine(" To App " + msg); } } public class MyApp { static void Main(string[] args) { SessionSettings settings = new SessionSettings(args[0]); IApplication myApp = new MyQuickFixApp();
You will also need to create a config for your program, right-click on the file explorer on the left in VSCode and select a new file. Call this file config.cfd. Copy the following code into this file replacing your SenderCompID and TargetCompID.
[DEFAULT] ConnectionType=initiator ReconnectInterval=2 FileStorePath=store FileLogPath=log StartTime=00:00:00 EndTime=00:00:00 UseDataDictionary=Y DataDictionary=FIX44.xml SocketConnectHost=fix-marketdata.tradermade.com SocketConnectPort=8003 LogoutTimeout=5 ResetOnLogon=Y ResetOnDisconnect=Y FileStorePath=incoming FileLogPath=outgoing [SESSION] # inherit ConnectionType, ReconnectInterval and SenderCompID from default BeginString=FIX.4.4 TargetCompId=YOUR_TARGET_COMP_ID SenderCompId=YOUR_SENDER_COMOP_ID HeartBtInt=30
Once you have created these files you can click ctrl+s to save them.
Now we can run the program, In the terminal type the following command.
dotnet run ./config.cfg
The program should connect to the server and you will see the live prices for the symbols you have requested.
From App 8=FIX.4.49=12535=S34=249=targetCompID51155486552=20230109-15:59:44.13456=senderCompID51155486555=GBPUSD117=1132=1.21915133=1.2191310=251 From App 8=FIX.4.49=12535=S34=349=targetCompID51155486552=20230109-15:59:45.14056=senderCompID51155486555=GBPUSD117=1132=1.21913133=1.2191110=246 From App 8=FIX.4.49=12535=S34=449=targetCompID51155486552=20230109-15:59:45.14856=senderCompID51155486555=GBPUSD117=1132=1.21913133=1.2191210=000 From App 8=FIX.4.49=12535=S34=549=targetCompID51155486552=20230109-15:59:46.14056=senderCompID51155486555=GBPUSD117=1132=1.21914133=1.2191110=250 From App 8=FIX.4.49=12535=S34=649=targetCompID51155486552=20230109-15:59:46.15256=senderCompID51155486555=GBPUSD117=1132=1.21916133=1.2191210=001