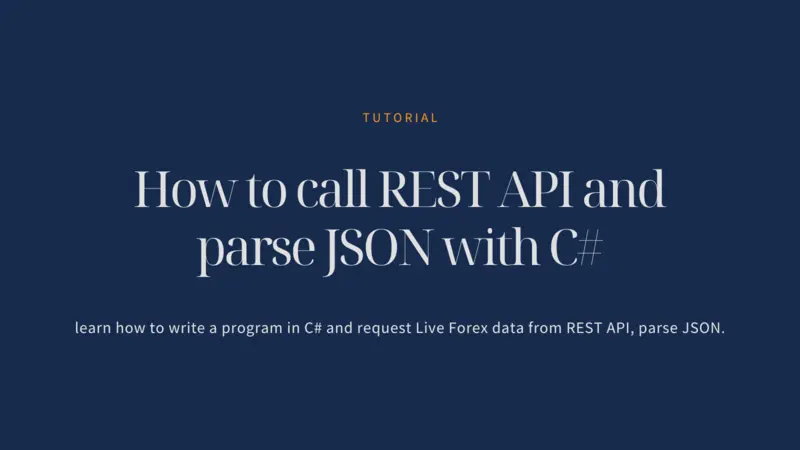
Forex REST API with C#: How To Parse JSON
30 April 2021
This tutorial guides you to set up your Visual Studio Code environment and write a program to request Live Forex data from TraderMade's REST API, parse that data, and output it to the console. If you already have your visual studio setup, you can skip the next section of this tutorial and jump to the coding section.
You can download a version of the code pre-populated with your API key here in our C# REST Example. This tutorial covers the live endpoint but could be adapted to work for any endpoint with a few minor adjustments.
First Up, We need to set up the environment.
Firstly, download and install .NET Core SDK and then install Visual Studio Code. We should set up and install the extension for c# to work in Visual Studio Code. Open the visual studio code, select View->Extensions in the search box, and enter C#. There are many extension options, but we will use the OmniSharp one for this example. Click the install button.
Initialise you project
Now we can build the project to pull the live Forex data - In windows explorer, create a new directory in your location of choice. You can call this by any name. We will name this "crest" as this is a C# REST Example. Now in VSCode, click the explorer tab and select the folder you just created.
Once this folder is loaded into VSCode, we need to initialise a .net skeleton - this will create a project definition file (.csproj) and the main file (.cs) into which we write our code.
Click View->Terminal and type the following command:
Dotnet new console
As standard, you will get a "Hello World" example. You may also get prompted to download any other assets needed to run the program. Select "Yes."
Install the NuGet
For this project, we need to install a third-party NuGet to help with JSON parsing. First, we need to install the NuGet package manager. Select the extensions tab, enter NuGet into the search box, and click install.
Now we have the NuGet package manager installed. We can install our helper libs. Press "F1" to bring up the VS Command pallet. Now we can type NuGet, and you will see the command "NuGet Package Manager Add Package."
This will then prompt for a package name. We will use "Newtonsoft.Json." Type this, and press enter to select the matching package and then the latest version.
Get your API key
The last thing you need for this program is your TraderMade API Key. If you do not have one, register from our signup page. Then you can copy it from your dashboard once you log in.
Ok, Let us write some code.
First, we need to add some using statements:
- The first is the standard system,
- The second System.Net.Http is for the call to the REST server, and
- The 3rd Newtonsoft.Json is a helper lib for parsing the JSON data returned.
using System; Using System.Net.Http; Using Newtonsoft.Json;
Now we create an HttpClient for our call to the server and make a call to the REST service for live data.
HttpClient client = new HttpClient(); HttpResponseMessage response = await client.GetAsync("https://marketdata.tradermade.com/api/v1/live?currency=EURUSD,GBPUSD&api_key=ENTER_YOUR_API_KEY"); response.EnsureSuccessStatusCode(); var responseBody = await response.Content.ReadAsStringAsync();
We are passing C# jsonobject as a parameter in the code, and getting JSON string containing our data as output. JSONserializer class converts any format of data into JSON.
Now we can use Newtonsoft to parse this.
The data returned from the server contains request information and then an array of market data. So, we need 2 classes to parse the returned data.
1) The Currency
2) Details class
public class CurrencyDetails { public string endpoint { get; set; } public quotes[] quotes { get; set; } public string requested_time { get; set; } public long timestamp { get; set; } }
The quote class;
public class quotes { public double ask { get; set; } public double bid { get; set; } public string base_currency { get; set; } public double mid { get; set; } public string quote_currency { get; set; } }
Once these classes are defined, we can parse the data into an easy-to-use JSON object. In the following code, we parse the data, iterate through the result, and output the data for the quotes.
Some users may prefer the output data in a much simplified manner than JSON. To convert JSON, they may need to deserialize the JSON. This could be a practical approach to get the output in a simplified format. JSONdeserialize class converts a JSON string into other formats.
var result = JsonConvert.DeserializeObject(responseBody); foreach (var item in result.quotes) { Console.WriteLine(" Result: " + item.base_currency + "/" + item.quote_currency + " " + item.mid); }
And now we can put it all together.
using System; using System.Net.Http; using Newtonsoft.Json; namespace crest { class Program { static async System.Threading.Tasks.Task Main(string[] args) { HttpClient client = new HttpClient(); HttpResponseMessage response = await client.GetAsync("https://marketdata.tradermade.com/api/v1/live?currency=EURUSD,GBPUSD&api_key=ENTER_YOUR_API_KEY"); response.EnsureSuccessStatusCode(); var responseBody = await response.Content.ReadAsStringAsync(); var result = JsonConvert.DeserializeObject<CurrencyDetails>(responseBody); foreach (var item in result.quotes) { Console.WriteLine(" Result: " + item.base_currency + "/" + item.quote_currency + " " + item.mid); } } public class CurrencyDetails { public string endpoint { get; set; } public quotes[] quotes { get; set; } public string requested_time { get; set; } public long timestamp { get; set; } } public class quotes { public double ask { get; set; } public double bid { get; set; } public string base_currency { get; set; } public double mid { get; set; } public string quote_currency { get; set; } } } }
And that's it! You now have a c# program that can request live forex rates from the TraderMade REST service.