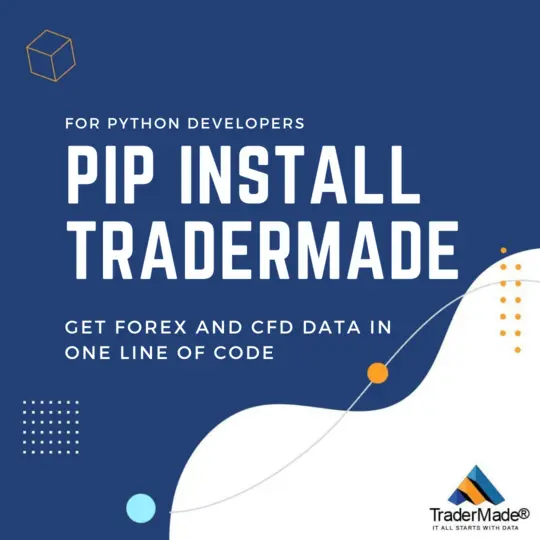
Get Forex and CFD Data with Python
27 April 2021
If you are a Python fan and SDK (Software Development Kit) enthusiast looking for forex data, look no further. I am introducing TraderMade Python-SDK, which will make your life much simpler concerning getting live and historical forex rates.
So let us begin.
As the first step, register and log in to get your API key from your dashboard. It takes seconds and is free for up to 1000 requests every month. Once you have an API key, note it in a safe place.
You can also follow our Python SDK tutorial video:
The second step is to install SDK, which is also simple. Just run the following command from the terminal (python 3 is required).
pip install tradermade
You can alternatively visit PyPI for more info. Once you have tradermade installed, open an IDE and run the following.
import tradermade as tm # set api key tm.set_rest_api_key("api_key")
You can now set the API key that will enable you to get data.
Live Forex Data
#get live data tm.live(currency='EURUSD,GBPUSD',fields=["bid", "mid", "ask"]) # returns live data - fields is optional
Running the above command gets you data. You can select what you want from the fields. If you only need the bid, provide
fields=[“bid”] and so on.
To obtain currency codes, just run the following:
#get currency codes tm.currency_list() # gets list of all currency codes available add two codes to get code for currencypair ex EUR + USD gets EURUSD
Historical Data
If you need historical forex data, then provide the date and the currency pairs you need the OHLC data for, and the SDK will return data for all those pairs for that date.
#get historical data tm.historical(currency='EURUSD,GBPUSD', date="2011-01-20",interval="daily", fields=["open", "high", "low","close"]) # returns historical data for the currency requested interval is daily, hourly, minute - fields is optional
To request granular data, change the date parameter to “YYYY-MM-DD-HH-MM” format, and you will get granular data as shown below.
Timeseries Data
If you are looking for timeseries analysis or plot data on a chart, then the timeseries function is the best. Provide a start and end date and interval you are looking for, as shown below. The daily endpoint only provides one year max of daily data per call, so to get data for more than a year, we can loop over the request, as shown below.
# get timeseries data import pandas as pd df = pd.DataFrame() for i in range(2011, 2021): x = tm.timeseries(currency='EURUSD', start=str(i)+"-06-17",fields=["open", "high", "low","close"], end=str(i+1)+"-06-16") df = df.append(x) df = df.drop_duplicates() df # returns timeseries data for the currency requested interval is daily, hourly, minute - fields parameter is optional (you can select ["close"] if you just want close prices)
The above example will provide a ten-year data set. However, for hourly and minute granularity, a single call can extract all data needed. The hourly timeseries are available for up to 2 months and minutes for up to 2 days. You can pass the period parameter if the minute interval is selected, default is 15.
# get timeseries data tm.timeseries(currency='EURUSD', start="2021-04-20",end="2021-04-22",interval="hourly",fields=["open", "high", "low","close"]) # returns timeseries data for the currency requested interval is daily, hourly, minute - fields parameter is optional (you can select ["close"] if you just want close prices)
If you want to request data for multiple currencies, then fields must be set to [“close”], as shown below.
tm.timeseries(currency='EURUSD,GBPUSD', start="2021-04-26",end="2021-04-27",interval="minute",fields=["close"],period=15) # returns 15 min bar for two currencies - you may need to adjust date to two days back or function will return an error that only two days of data is allowed for minute interval.
As you can see, compared to using an API directly, this makes it easier to get forex data. For more info, visit the TraderMade docs page. Hope this helps. If you have any requests or questions, please let us know.